Creating a web interface - KeplerGL
In this tutorial, we will look at how to connect the open-sourced KeplerGL project to the Tracking Stream API in a browser.
caution
#
Source codeYou can find and download the source code for this example here.
#
Setting up ReactFor the sake of simplicity, we are gonna use the create-react-app utility.
Simply start by the following commands:
We use the --template redux
flag to set up a Redux store in our app, since KeplerGL depends on Redux to work properly.
You should now have a React application running at http://localhost:3000.
#
Setting up KeplerGLWe can now add KeplerGL to our application:
In our main App.js
file, we can then import at the top
Let's also install react-virtualized, and use its <Autosizer>
component which we'll use to dynamically resize our KeplerGL DOM element.
We can now import it, clean up the previous default code, and set up our KeplerGL map component.
To make KeplerGl work, we need to setup the appropriate Redux state manager and middlewares:
As you can see, we also did set a several configuration parameters in the initial state of the reducer of KelperGL.
#
Connecting to the Tracking Stream endpointWe can now set up our Tracking Stream API call and data parsing code and then display it with KeplerGL. If you want to learn more about reading streams using the Javascript fetch
API, you can find more resources here.
Let start by creating a new function in our component:
#
Let's break down what was done here- First, we create a function
extractJSON
that will extract all the JSON objects contained in the received lines and split them into an array of JSON strings using a Regular expression. - Second, we create an
async
function that calls our endpoint and get a stream reader (usinggetReader()
) from the obtained response. - We infinitely loop through our stream reader, reading our values as we go, extracting their JSON content, and replacing old entries sharing the same
icao_address
, or creating new entries if theicao_address
wasn't found. - We
dispatch
the Redux actionaddDataToMap
(using theuseDispatch
hook) from KeplerGL, updating the Redux store in the process.
#
Kepler data configurationWe are almost done with our example, the last thing to do is creating the configuration file imported as keplerConfig
.
This configuration will indicate to KeplerGL what fields are present in our data, and their specificities such as data types, colors, tooltip options, etc ...
Simply create a new file src/keplerConfig.js
, and set the configuration variables:
#
Let's break down what was done here- We created the
dataFields
array, which lists the fields that will appear in each Kepler entry, with their respective types. - We created the
tooltipFields
array, which lists which fields will be visible when hovering over a data point. - We created the
initialConfig
object, which details the initial configuration for our Kepler layers and datasets.
#
Finishing upAll we need to do now is call our listenToStream()
function at render time. We'll do that by adding a useEffect
with the parameter []
, which will call the function only once on the first render.
Also strict mode of react should be prevented because it renders KeplerGL map twice.
Make sure your index.js looks like following, you need to remove React.StrictMode
component:
🎉 We now have a basic functional example of a KeplerGL map displaying the Tracking Stream data. For reference, it should look like that:
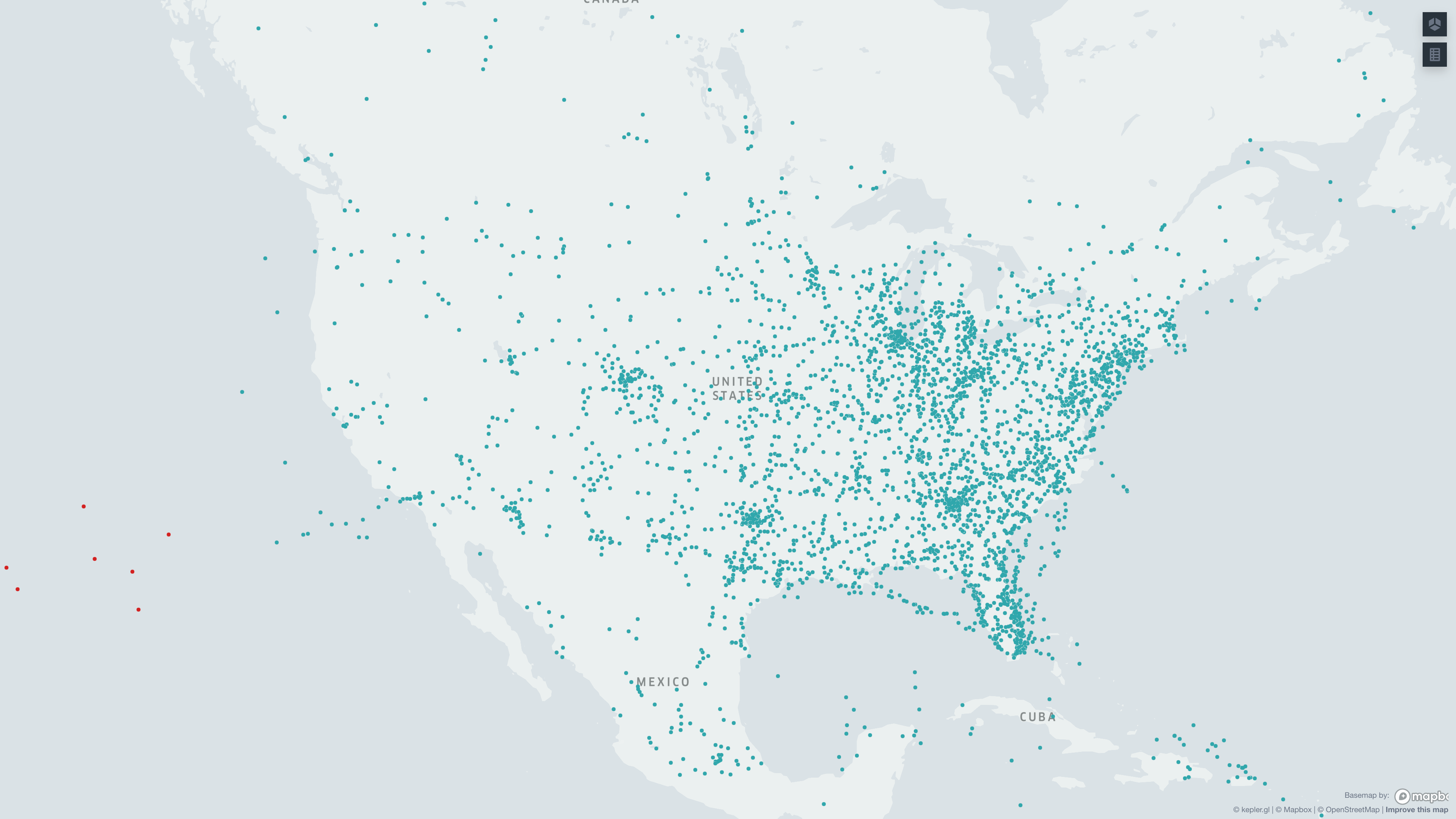
Next steps
We could improve that example by adding a button to close/open the connection, display some statistics about what is currently being displayed among many other possible improvements.